To eliminate unqualified candidates in a programming job, they are given a basic programming task called fizz buzz test. While the fizz buzz may not be designed as the final examination, it serves its purpose of helping hiring officers to know the potential candidates.
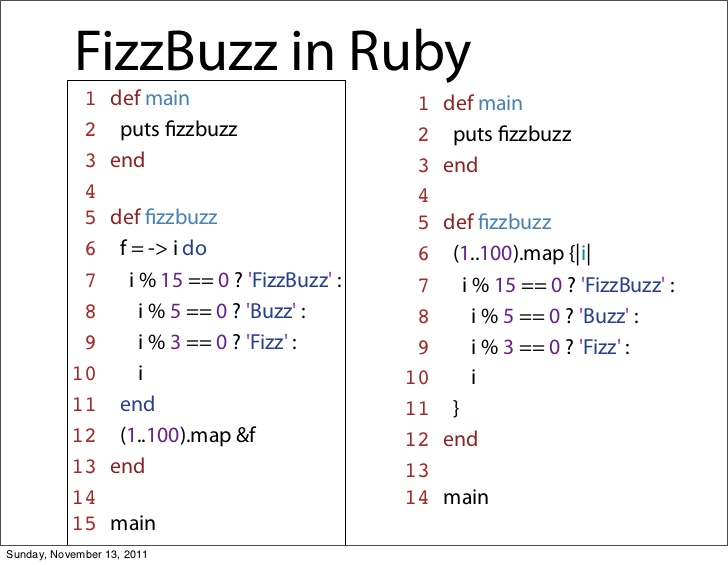
Don’t get us wrong for the actual program you would be asked to write differs from one company to another. However, the most popular may observe these: Create a program that prints out the numbers 1 to 100, with the multiples of 3 print Fizz; with the multiples of 5 print Buzz; and multiples of both 3 and 5 print FizzBuzz.
- The Loop (Writing a Program that Prints Out Numbers 1 to 100)
First thing you must regard is how to iterate over the numbers of 1-100 and in the case of you translating it in the language of Ruby, the best decision you can do is to pick the most native format or idiomatic approach.
Least Ruby-like statement:
i = 1 while 1 <= 100 do # Do fizz buzz end
Programmers adept in C, C++ and Java may try to do this which is just fine but not as native in the Ruby language. Experts who see this will immediately conclude that the candidate may be not be a pro when it comes to Ruby.
for i in 1..100 do # Do fizz buzz end
Now, using a for loop definitely tells your hiring officers that you don’t know Ruby “that” well.
1.upto(100) do|i| # Do fizz buzz end
Yes, the upto method obviously tells that you are good in Ruby since it exposes clearly what this code is doing. Despite having the issue of difficulty to separate the magic numbers, 1 and 100, from the function of the code, this is certainly a good choice of a loop.
On the other hand, you can utilize Ruby’s primitive type for dealing with ranges of numbers. This may be needed should a refactor may be needed to change the range—for instance—from 10 to 50. The presence of a range to drop in will help you a lot in simplifying the code modification.
(1..100).each do|i| # Do fizz buzz end
You simply expressed the most idiomatic loop in the Ruby language.
- Divisibility (Testing If the Number is Divisible by 3 or 5)
To test this, you must have an integer division since you will always get a quotient (integer result) and remainder (modulus). For instance, when dealing with the integer math 10/3 will result the quotient 3 and a remainder of 1. If the remainder is 0, then the divisor was evenly divided (since there’s no remainder present) and is hence, divisible. In the Ruby, the modulo operator is %, so you can simply test the divisibility of n by m by saying n % m==0.
Using modulo method, however, brings slightly clearer results. For a simple program, you can leave the modulo operator alone.
(1..100).each do|i| if i % 3 == 0 puts “Fizz” end end
Now, we have to do the extracting of a method by using the Ruby-style Fixnum class. You can use this since it is relatively simple program with limited scope:
class Fixnum def divisible_by?(n) modulo(n) == 0 end end (1..100).each do|i| if i.divisible_by?(3) puts “Fizz” end end
Remember that since this is an idiomatic Ruby, a return statement is not explicitly required.
- Control Structures (Final: Printing Fizz, Buzz or FizzBuzz)
Just choose the most obvious solution of all:
class Fixnum def divisible_by?(n) modulo(n) == 0 end end (1..100).each do|i| if i.divisible_by?(3) && i.divisible_by?(5) puts “FizzBuzz” elsif i.divisible_by?(3) puts “Fizz” elsif i.divisible_by?(5) puts “Buzz” else puts i end end
You can also regard this option to avoid the dryness of the Fizz and Buzz strings:
class Fixnum def divisible_by?(n) modulo(n) == 0 end end (1..100).each do|i| output = [i] output << “Fizz” if i.divisible_by?(3) output << “Buzz” if i.divisible_by?(5) output.shift if output.length > 1 puts output.join end
This should work by building a wide range of things you want to print. You can begin with the number and add Fizz and Buzz, depending on the divisibility. Just bear in mind that Fizz and Buzz are not repeated; thus, it is simpler and easier for it to refactor.